What is auto keyword in C++ 11 ?
December 12, 2021
C++
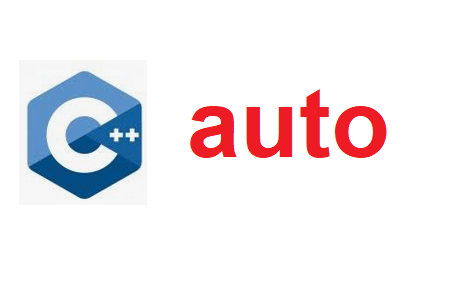
Using auto specified we can declare any variable without specifying it’s data type. Variable will automatically determine it’s data type using value assign to variable.
auto Int_Var = 1;
auto Char_Var = 'P';
auto Str_Var = "Hello";
cout<< Int_Var <<endl; // Output Integer value: 1
cout<< Char_Var <<endl; // Output Character value: P
cout<< Str_Var <<endl; // Output String value: Hello
We can also store Lambda function inside auto variable.
#include <iostream>
using namespace std;
auto AddFunc = [](int a, int b)
{
return (a + b);
};
int main()
{
cout << AddFunc(1,2) << endl; // output : 3
return 0;
}
Main advantage of auto comes with types that are long to write.
#include<iostream>
#include<map>
using namespace std;
int main()
{
map<int,string> MapObj;
MapObj.insert(pair<int,string>(1,"One"));
MapObj.insert(pair<int,string>(2,"Two"));
MapObj.insert(pair<int,string>(3,"Three"));
//map<int,string>::iterator it = MapObj.begin();
auto it = MapObj.begin(); // benefit or auto
while(it != MapObj.end()){
cout<<it->first<<"::"<<it->second<<endl;
it++;
}
return 0;
}
Once we have initialized the auto variable then you can change the value but you cannot change the type.
auto MyVar = 10;
cout<<MyVar<<endl;
MyVar = 20;
cout<<MyVar<<endl;
MyVar = "HELLO";
//error: invalid conversion from ‘const char*’ to ‘int’
auto cannot be left uninitialized. We must initialize it with value.
auto MyVar;
//error: declaration of ‘auto MyVar’ has no initializer
In C++11, you can now put the return value at the end of the function declaration, substituting auto for the name of the return type.
auto Add(int a, int b) -> int
{
return (a+b);
}
int main()
{
auto MyVar = Add(1,2);
cout<<MyVar<<endl; // Output: 3
return 0;
}